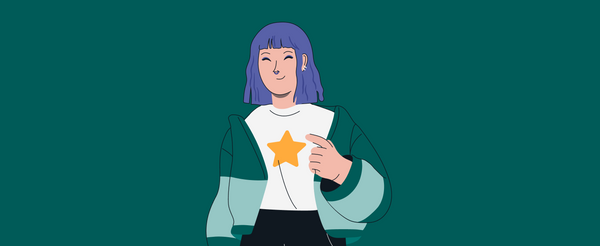
Hotel Legacy System Modernization: Key Steps and Challenges to Enhance Operational Efficiency
Running a hotel with outdated systems is frustrating. Slow check-ins, lost reservations, and staff drowning in manual data entry. These problems hurt your guests and your bottom line.