R&D project: GPS Tracking App. Experiments in Android
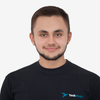
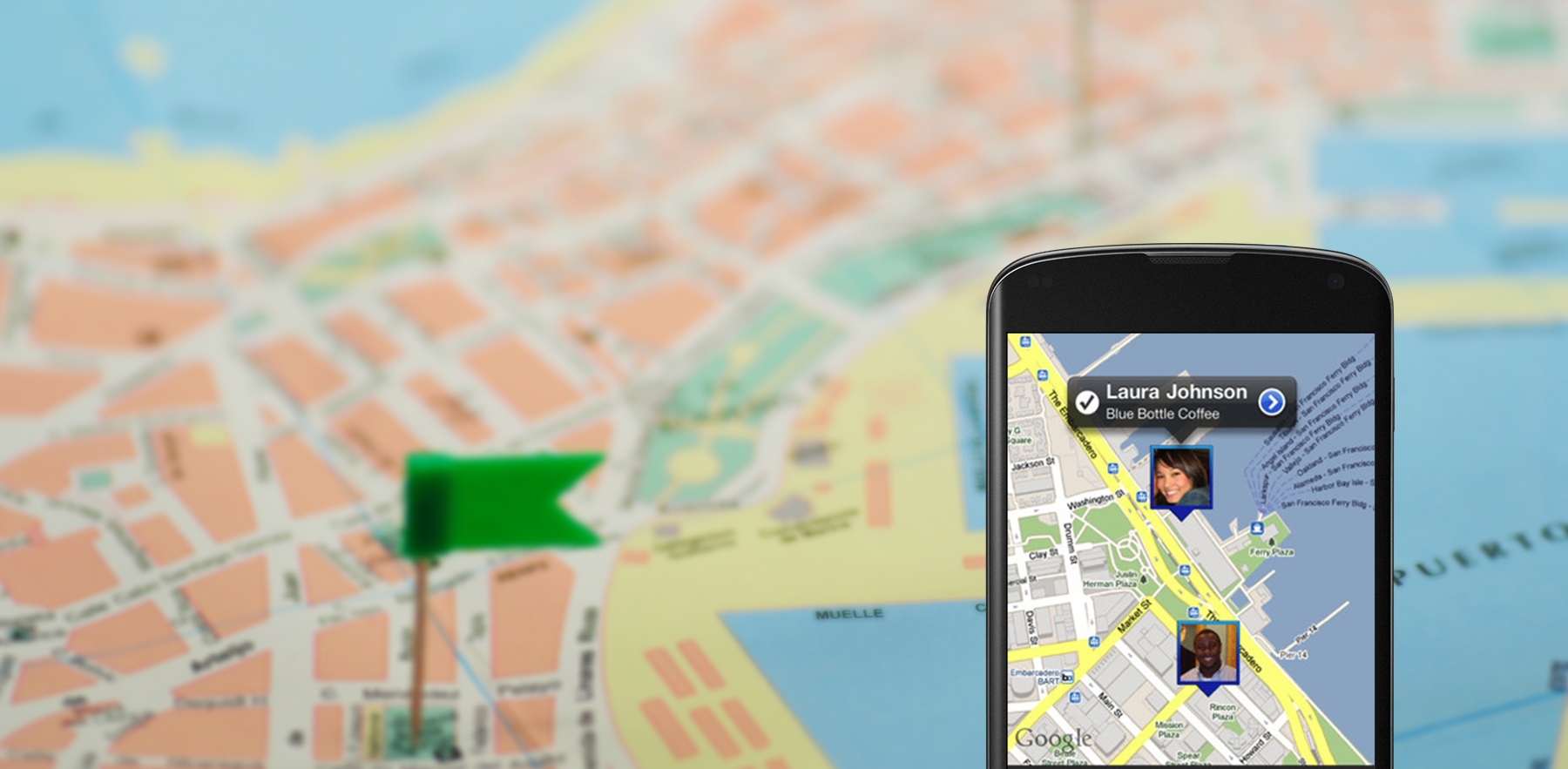
Would you like to convert your mobile device into a GPS tracking device? Use it in a social way, track your friend's location in real time, get notifications when some of them are walking nearby and don’t miss the chance to hang out together? In our R&D project
Would you like to convert your mobile device into a GPS tracking device? Use it in a social way, track your friend's location in real time, get notifications when some of them are walking nearby and don’t miss the chance to hang out together? In our R&D project concerning location tracking apps, we investigated different mechanics as to how to provide this service in a user-friendly way with a minimal battery usage.
Lots of different great ideas based on knowing current the user’s location are already being probed. Having a profitable background, we also decided to contribute in this area and create a product to see how a mobile tracker with map location works in real life.
Our first goal was to learn how to track a user’s location. Tracking means that we have to save a person’s current location on the onset of a person’s change in global positioning and then again at some time since the last updated has passed. Also, we had to investigate the behavior of the Android location API services with different configurations (frequency of location updates and user displacement) and the impact of tracking on battery drainage. Once all these priorities have been set, we have to implement a synchronization mechanism to send new location data to the server. Our location tracking app is free and available for everyone who is willing to use their cell phone as a GPS tracking device.
First of all, I want to state that a user’s location is a very valuable data for different purposes. For instance, track my cell phone to know where your child is or which locations your employee had visited 4 hours ago. The way you use this location depends on problems you are trying to solve. Current smartphones give us a possibility to make location tracking easy and most importantly, without any additional devices. Of course, there are some challenges faced when obtaining user location from a mobile device and we have to be attentive to them and do our best to solve location-related problems. There are several reasons provided by Google, as to why a location reading (regardless of the source) can contain errors and be inaccurate. Some sources of error in the user location may include:
- Multitude of location sources;
- GPS, Cell-ID, and Wi-Fi can provide a clue to user's location. Determining which one to use and trust is a matter of trade-offs in accuracy, speed, and battery efficiency;
- User movement;
- As the user location changes, you must take this into account by re-evaluating it more often;
- Varying accuracy;Location estimates coming from each location source are not consistent in their accuracy. A location obtained 10 seconds ago from one source might be more accurate than the current location that was obtained from another source or better yet, the same source.
Android OS has 2 different well-known APIs to get user’s location: Location Manager from android.location and Location APIs from Google Play Services. Taking into account our previous experience working with Location manager, we’ve chosen Google Play Services location APIs as the one to utilize. It is easy to configure, provides good results, is flexible and easy to use for different problems. All you need is to make sure that the appropriate version of Google Play services is installed on a device.
Generally, I think Google Play services is a great part of Android ecosystem and everybody has to take advantage of it.
So, we started the development. Frankly speaking, first steps were easy because Google provides very good samples of how to set up Google Play Services and how to start using geolocation API. The model of the way location APIs work is simple: you have to initialize google API client object and subscribe to receive location updates. You also have to configure an instance of a location request object with parameters you want and to provide this object while subscribing to receive location updates. Really simple.
Google API client initialization:
GoogleApiClient googleApiClient = new GoogleApiClient.Builder(context)
.addConnectionCallbacks(connectionCallback)
.addOnConnectionFailedListener(
connectionFailedListener)
.addApi(LocationServices.API)
.build();
Start location updates:
LocationRequest locationRequest = createLocationRequest();
LocationServices.FusedLocationApi.requestLocationUpdates(
googleApiClient, locationRequest, locationListener);
Location request encapsulates all the data that the location service needs, in order to provide us with accurate location updates. Generally, it defines the quality of location updates by setting different parameters such as priority, interval, smallest displacement, etc. For instance, let’s set location request priority, and for this field, we’ll plug in a bunch of predefined constant values. By setting request priority, we “tell” location service the level of accuracy that is expected: is it a “city” level or “block” level. It is very important to understand your location “needs” and choose the right priority. It will help to resolve your specific problem and to minimize battery drainage. Also, it’s possible to specify the number of location updates you need, and thus, it too can be very useful.
Location request object configuration:
public LocationRequest createLocationRequest() {
LocationRequest locationRequest = new LocationRequest();
locationRequest.setInterval(5 * 60 * 1000);
locationRequest.setPriority(
LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY);
locationRequest.setSmallestDisplacement(100);
return locationRequest;
}
Taking into account all the predispositions mentioned above, we developed our application. It has a very simple UI design along with straightforward functions. You can choose one of the location requests specified and start/stop location tracking.
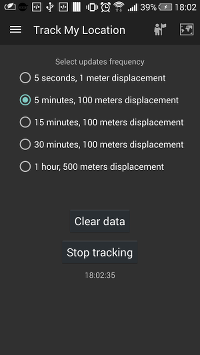
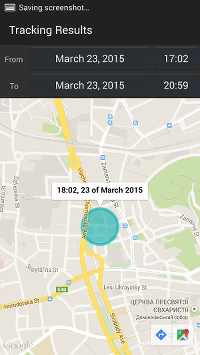
We implemented a service which is a listener subscribed to receive location updates. As you know, Android service provides a possibility to perform tasks in the background, and that’s what we need. Once location changes are received via appropriate callback, they are stored into the database. The synchronization of the data with the server appears every hour. Synchronization scheduling is done using Android AlarmManager.
So, let’s analyze some results from tracking.
To represent tracking results, the website with a map for every user and an activity map within a mobile app was created. We tried different combinations, such as the frequency of location update and the priority in requesting location. When we were tracking a location every 5 minutes with “PRIORITY HIGH ACCURACY” the result was as expected - accurate location updates were received and high battery usage was detected. Probably, some set of problems really need very accurate location, thus you have to be ready to charge the battery more frequently.
Using PRIORITYBALANCEDPOWER_ACCURACY with 100 m as the smallest displacement and 30 minutes interval showed much better results - we still were receiving location updates that we needed, but we didn’t notice high battery usage. Hence, we proved that there is a correlation between location update accuracy and battery consumption - the more accurate you want the location update to be, the more battery life you should be ready to drain. I think it’s obvious.
Here you can see some battery statistics:
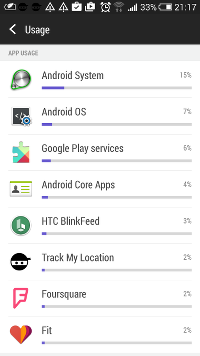
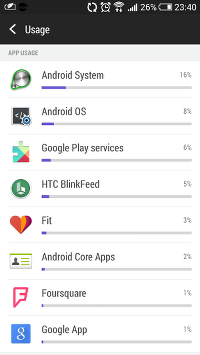
I’ve launched the location tracking app at 7 PM. As you can see, our Android GPS position reporting app consumed 2% of total battery life used at 9:21 PM, and at 11:40 PM it disappeared from the applications that put a toll on battery life. During the time frame, from 7 PM till 11:40 PM, 8 locations were tracked and sent to the server while battery usage was monitored to be normal throughout that time period.
So having such a background in location tracking, we decided to wrap it into an application. And we’ve figured out the following idea - it would be great to know if one of your friends or acquaintances is not far away from you. Your smartphone will help you to get this done. The app grabs your location data as well as your friend’s location data. Then, the data is synchronized with the server and the server searches for some matches - when your location is close to your friend’s, you will receive a push notification with “Hi!” message and with a name of your friend who is near you. For push notifications we use Parse service - it is very easy to setup and to start using it. Also, the application has a Facebook login for grabbing and pulling up the information about the user.
We called this application “Hi!”. You can use this location tracking app for meeting friends and having a great time together. Cool idea, don’t you think?
Another interesting area that we were examining is geofencing. Geofence itself is an area defined by the coordinates of its center and a radius. It is great to know if a user entered or left some area or geofence. This information can be managed in different ways, and Android is here to help us solve the problem. Geofencing API is a part of Google Play Services location APIs. The way it works is very close to location tracking - you have to subscribe to receive geofencing event using a list of geofences. Each geofence is defined by a latitude and longitude of its center and radius. You have to keep in mind that only 100 geofences can be active at once on a single device. The result is received by IntentService you define. The data which you receive contains all the necessary information about what has happened: the event type (entered or exited the geofence), geofence name. The rest depends on you-you can perform the corresponding action you want, depending on the event that appeared.
Our implementation has a UI part to define geofences.
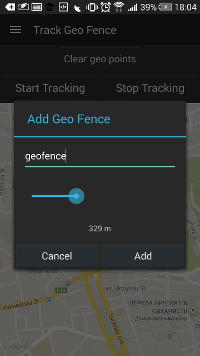
Once you predefined them (the values), you can start with android location tracking. When you entered or exited some geofence notification appears in notification bar and it shows an event type and geofence’s name.
We were testing our application using a geofence with a radius equal to 100 meters. Actually, it is not a big value. We noticed that sometimes a callback about you entering or leaving geofence does not appear immediately, and it might even take up to 2 - 5 minutes. There are some factors causing it: a small radius of a geofence and the way API updates the location. Also, location accuracy has its impact on it. So, generally, geofence API callbacks are sent correctly and they bring actual information about some area that the user has entered or exited.
Working with Android location APIs brought a lot of interesting moments. It was very cool to test it in real conditions walking on the street or going somewhere while in a car. Of course, the accuracy of location updates could be better, nevertheless, it is still enough for existing problems. Actually, your smartphone is not responsible to deliver precise user’s location because it’s not the smartphone’s main function.
To conclude, GPS location tracker app “Hi!” is just a small R&D project from the TechMagic team that was released to discover how location services of your cell phone can be useful in real life. With its existent features, you can track a friend’s location, get notifications when they appear nearby and meet up with them to have a great time together. It’s just an MVP, but on the next stage of the development, more complex social features will be implemented in order to create the best phone tracking app for Android based on social goals. Everything is available at https://github.com/techmagic-team/track-my-location.
Looking for more cool mobile apps with geolocation? Check out our portfolio at https://www.techmagic.co/